Today, you are going to learn how to display 24 hours TimePicker upon clicking on edit text in Android Studio.
Let me talk little more about TimePicker before I jump into the coding section.
In Android, TimePicker is a widget for selecting the time of the day, in either AM/PM or 24-hour mode.
Here, I am going to show you 24-hour mode TimePicker.
Let’s begin in the coding section.
Step 1: XML File
Create your xml file with edittext. See an example below.
<EditText
android:id="@+id/editTimePicker"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:autofillHints="@string/hint_pick_up_time"
android:focusable="false"
android:background="#FFFFFF"
android:hint="@string/hint_pick_up_time"
android:inputType="time"
android:textColor="@color/black"
android:textSize="15sp" />
Don’t forget to add the following code in your xml edittext. I have already added.
android:focusable="false"
Step 2: strings.xml
<resources>
<string name="hint_pick_up_time">hh/mm</string>
</resources>
Step 3: Jave Code
Edittext etTime = findViewById(R.id.editTimePicker);
Create your edittext onClickListerner. Add the below code to function edittext click listerner.
//Time Picker Dialog
etTime.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Calendar myCalendar = Calendar.getInstance();
int hour = myCalendar.get(Calendar.HOUR_OF_DAY);
int minute = myCalendar.get(Calendar.MINUTE);
// time picker dialog
TimePickerDialog mTimePicker;
mTimePicker = new TimePickerDialog(Home.this, new TimePickerDialog.OnTimeSetListener() {
@Override
public void onTimeSet(TimePicker timePicker, int selectedHour, int selectedMinute) {
etTime.setText( selectedHour + ":" + selectedMinute);
}
}, hour, minute, true); //Yes 24-hour time mode
mTimePicker.setTitle("Select Time");
mTimePicker.show();
}
});
Output:
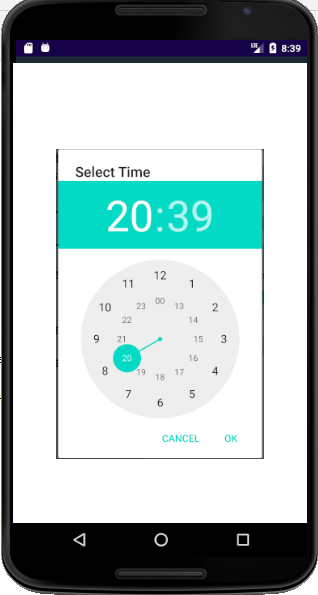
AM/PM mode in clock
To display your clock mode into AM/PM 12-hour time, then change the below highlighted code from “true” to “false“.
//Time Picker Dialog
etTime.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
Calendar myCalendar = Calendar.getInstance();
int hour = myCalendar.get(Calendar.HOUR_OF_DAY);
int minute = myCalendar.get(Calendar.MINUTE);
// time picker dialog
TimePickerDialog mTimePicker;
mTimePicker = new TimePickerDialog(Home.this, new TimePickerDialog.OnTimeSetListener() {
@Override
public void onTimeSet(TimePicker timePicker, int selectedHour, int selectedMinute) {
String time = selectedHour + ":" + selectedMinute;
SimpleDateFormat fmt = new SimpleDateFormat("HH:mm");
Date date = null;
try {
date = fmt.parse(time );
} catch (ParseException e) {
e.printStackTrace();
}
SimpleDateFormat fmtOut = new SimpleDateFormat("hh:mm aa");
assert date != null;
String formattedTime=fmtOut.format(date);
etTime.setText(formattedTime);
}
}, hour, minute, false);//No 24 hour time
mTimePicker.setTitle("Select Time");
mTimePicker.show();
Output:
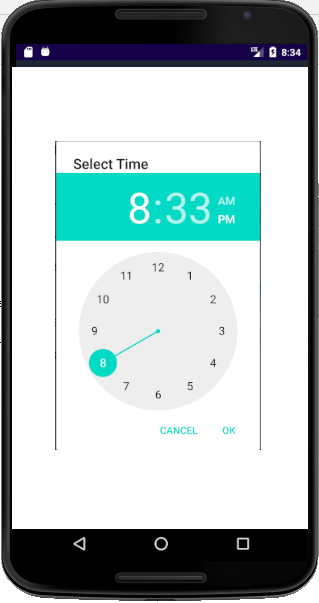
You may interest on the following topics:
- How to implement searchView widget in android Studio through Recycleview?
- How to create an expandable listview in Fragments in Android Studio?
- How to create an expandable list view in Android Studio?
- How do I check Intel Virtualization Technology is enabled or not in Windows 10?
- Plugin ‘Android WiFi ADB’ is compatible with IntelliJ IDEA only because it doesn’t define any explicit module dependencies
- Could not install Gradle Distribution from ‘https://services.gradle.org/distributions/gradle-6.5-all.zip’
- How to solve “INSTALL_PARSE_FAILED_NO_CERTIFICATES” error in Android Studio?
- Android Studio Run/Debug configuration error: Module not specified.
- How to search in multiple nodes in Firebase Database?
- How to get the sum of child nodes in Firebase database?
- How to display website in an android app using WebView component?
- Android Layout Using ViewPager and Fragments
- How do I install Android Studio in Windows 10?
- How to display ListView in Fragments using ViewPagers?
- How to create a custom AlertDialog in Android Studio?
- How do I change the name under apps that display in google play store?
- Where does my database store in Android Studio?
- How to add google places autocomplete in Android Edittext?
- How do I convert dp into px in the android studio?
- What are the android screen background sizes?
- What are the sizes of the Android icon located inside the mipmap?
- How do I remember my android app signing key?
- How do I create a Toolbar in Android Studio?
- How to get Android Spinner Dropdown?
- error: package R doesn’t exist in android studio project?
- Firebase Email and Password registration for Android App
- How do I change the company domain name in Android Studio after creating a project?
- How do I make an existing Android Studio Project copy?
- How do I migrate an Android Studio 3.2 existing project to Android X?
- Step by step to insert both banner and interstitial ads in android apps.
- Android TimePicker upon clicking on edittext in Android Studio?
- Migrating to AndroidX Errors:
- How to popup date picker when clicking on edittext in Android Studio?
- AndroidX: ExampleInstrumentedTest.java Class Source code
- How to add user registration data into the Firebase database?
- Cannot find Symbol: ApplicationTestCase
- How do I use Android vector images in Android apps?
- How to create a new Android Virtual Device (AVD) in Android Studio?
- On SDK version 23 and up, your app data will be automatically backed up and restored on app install.
- App is not indexable by Google Search; consider adding atleast one Activity with an Action-View intent-filter.
- How do I style my button in Android app?
- How do I create drawable folder in Android Studio?
- How do I create new classes in Android Studio?
- How to create new android project tutorial?
- How to upgrade Android Studio 2.2.3 to new versions or Android Studio 3.5.3?
- error: Package R does not exist after renamed package name?