In this tutorial, I am going to show you how can you show a toolbar menu in Android. First, you need to understand the toolbar.
Toolbar is a ViewGroup that can be placed anywhere in your layout. By default, Android uses an action bar. You need to replace the action bar with a toolbar to use a toolbar.
You can easily replace Actionbar into Toolbar by using setSupportActionBar() method. The below code helps to replace ActionBar into ToolBar.
Toolbar mToolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(mToolbar);
See an image below. I am going to show you how you can achieve this type of toolbar output.
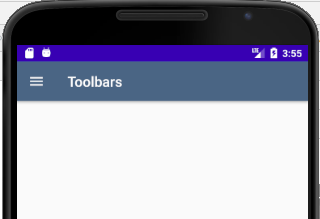
Let start from the beginning to create toolbar into Action bar.
Step 1: Add support library
To use the Toolbar you need to add supporting dependencies in your app’s build.gradle file.
Android -> Gradle Scripts -> build.gradle (Module:app)
implementation 'com.google.android.material:material:1.2.1' //design support library for AndroidX
Step 2: Change App Theme ActionBar
Here, you have to disable your App Theme ActionBar from your style.xml resource file.
Your style.xml resource file before disable AppTheme ActionBar
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
<style name="AppTheme.NoActionBar">
<item name="windowActionBar">false</item>
<item name="windowNoTitle">true</item>
<item name="android:statusBarColor">@android:color/transparent</item>
</style>
</resources>
After disable your style.xml App theme ActionBar
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
<style name="AppTheme.NoActionBar">
<item name="windowActionBar">false</item>
<item name="windowNoTitle">true</item>
<item name="android:statusBarColor">@android:color/transparent</item>
</style>
</resources>
Step 3: Design your Toolbar with icon and Toolbar title
Create a xml file and named as toolbar.xml. And add a menu icon and toolbar title “Toolbar”.
<?xml version="1.0" encoding="utf-8"?>
<androidx.drawerlayout.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/drawer_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
tools:openDrawer="start">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="@color/blue"
android:elevation="4dp"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light"
app:navigationIcon="@drawable/ic_baseline_menu_24"
app:title="Toolbar"/>
</LinearLayout>
</androidx.drawerlayout.widget.DrawerLayout>
Step 4: Toolbar Activity
Add the below code inside your activity OnCreate() method.
Toolbar mToolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(mToolbar); // Setting replace toolbar as the ActionBar
That’s it. Now run your program and see the output. A toolbar with menu icon and title will shown on the top of your app.
Donate to support writers
You may interest on the following topics:
- How to implement searchView widget in android Studio through Recycleview?
- How to create an expandable listview in Fragments in Android Studio?
- How to create an expandable list view in Android Studio?
- How do I check Intel Virtualization Technology is enabled or not in Windows 10?
- Plugin ‘Android WiFi ADB’ is compatible with IntelliJ IDEA only because it doesn’t define any explicit module dependencies
- Could not install Gradle Distribution from ‘https://services.gradle.org/distributions/gradle-6.5-all.zip’
- How to solve “INSTALL_PARSE_FAILED_NO_CERTIFICATES” error in Android Studio?
- Android Studio Run/Debug configuration error: Module not specified.
- How to search in multiple nodes in Firebase Database?
- How to get the sum of child nodes in Firebase database?
- How to display website in an android app using WebView component?
- Android Layout Using ViewPager and Fragments
- How do I install Android Studio in Windows 10?
- How to display ListView in Fragments using ViewPagers?
- How to create a custom AlertDialog in Android Studio?
- How do I change the name under apps that display in google play store?
- Where does my database store in Android Studio?
- How to add google places autocomplete in Android Edittext?
- How do I convert dp into px in the android studio?
- What are the android screen background sizes?
- What are the sizes of the Android icon located inside the mipmap?
- How do I remember my android app signing key?
- How do I create a Toolbar in Android Studio?
- How to get Android Spinner Dropdown?
- error: package R doesn’t exist in android studio project?
- Firebase Email and Password registration for Android App
- How do I change the company domain name in Android Studio after creating a project?
- How do I make an existing Android Studio Project copy?
- How do I migrate an Android Studio 3.2 existing project to Android X?
- Step by step to insert both banner and interstitial ads in android apps.
- Android TimePicker upon clicking on edittext in Android Studio?
- Migrating to AndroidX Errors:
- How to popup date picker when clicking on edittext in Android Studio?
- AndroidX: ExampleInstrumentedTest.java Class Source code
- How to add user registration data into the Firebase database?
- Cannot find Symbol: ApplicationTestCase
- How do I use Android vector images in Android apps?
- How to create a new Android Virtual Device (AVD) in Android Studio?
- On SDK version 23 and up, your app data will be automatically backed up and restored on app install.
- App is not indexable by Google Search; consider adding atleast one Activity with an Action-View intent-filter.
- How do I style my button in Android app?
- How do I create drawable folder in Android Studio?
- How do I create new classes in Android Studio?
- How to create new android project tutorial?
- How to upgrade Android Studio 2.2.3 to new versions or Android Studio 3.5.3?
- error: Package R does not exist after renamed package name?