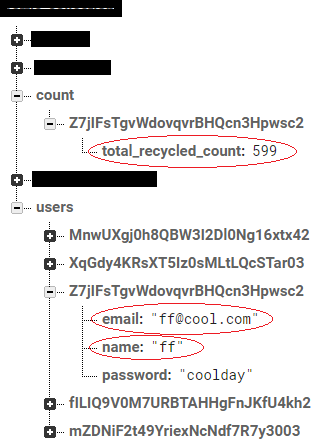
In this tutorial, I will show you how to get the value from multiple node and set value in your textview.
Here, we will get the value of total_recycled_count from count node and email and name from users node.
The solution is pretty simple. To get the value from two different nodes you need to query your database twice.
Code:
//Initialize variable
currentUser = mAuth.getCurrentUser();
if(currentUser != null) {
final String uid = currentUser.getUid();
FirebaseDatabase firebaseDatabase = FirebaseDatabase.getInstance();
DatabaseReference databaseReference = firebaseDatabase.getReference().child("count");
Query query = databaseReference.orderByChild(uid).equalTo(uid);
ValueEventListener valueEventListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
//test for null and validity
if(dataSnapshot.exists() && dataSnapshot.getValue()!= null) {
for(DataSnapshot ds : dataSnapshot.getChildren()) {
String totalRCount = Objects.requireNonNull(ds.child("total_recycled_count").getValue()).toString();
//set totalRecycledCount in a profile textview
profile_totalrecycledcount.setText(totalRCount);
DatabaseReference uidRef = firebaseDatabase.getReference().child("users").child(uid);
ValueEventListener eventListener = new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String name = dataSnapshot.child("name").getValue(String.class);
String email = dataSnapshot.child("email").getValue(String.class);
Log.d("TAG", name + " / " + email);
//set profile in result textviews
//Don't forget to initialize your profile_name and profile_email and get their xml reference, which is not shown in this code.
profile_name.setText(name);
profile_email.setText(email);
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {}
};
uidRef.addListenerForSingleValueEvent(eventListener);
}
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {}
};
databaseReference.addListenerForSingleValueEvent(valueEventListener);
}
Output:
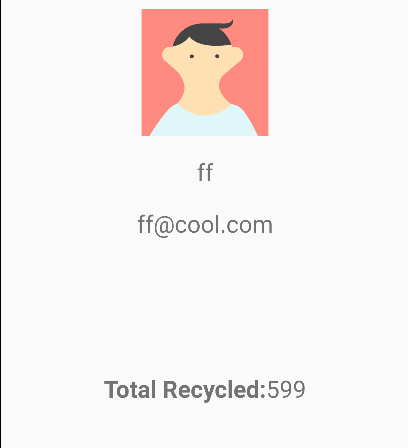
You may interest on the following topics:
- How to implement searchView widget in android Studio through Recycleview?
- How to create an expandable listview in Fragments in Android Studio?
- How to create an expandable list view in Android Studio?
- How do I check Intel Virtualization Technology is enabled or not in Windows 10?
- Plugin ‘Android WiFi ADB’ is compatible with IntelliJ IDEA only because it doesn’t define any explicit module dependencies
- Could not install Gradle Distribution from ‘https://services.gradle.org/distributions/gradle-6.5-all.zip’
- How to solve “INSTALL_PARSE_FAILED_NO_CERTIFICATES” error in Android Studio?
- Android Studio Run/Debug configuration error: Module not specified.
- How to search in multiple nodes in Firebase Database?
- How to get the sum of child nodes in Firebase database?
- How to display website in an android app using WebView component?
- Android Layout Using ViewPager and Fragments
- How do I install Android Studio in Windows 10?
- How to display ListView in Fragments using ViewPagers?
- How to create a custom AlertDialog in Android Studio?
- How do I change the name under apps that display in google play store?
- Where does my database store in Android Studio?
- How to add google places autocomplete in Android Edittext?
- How do I convert dp into px in the android studio?
- What are the android screen background sizes?
- What are the sizes of the Android icon located inside the mipmap?
- How do I remember my android app signing key?
- How do I create a Toolbar in Android Studio?
- How to get Android Spinner Dropdown?
- error: package R doesn’t exist in android studio project?
- Firebase Email and Password registration for Android App
- How do I change the company domain name in Android Studio after creating a project?
- How do I make an existing Android Studio Project copy?
- How do I migrate an Android Studio 3.2 existing project to Android X?
- Step by step to insert both banner and interstitial ads in android apps.
- Android TimePicker upon clicking on edittext in Android Studio?
- Migrating to AndroidX Errors:
- How to popup date picker when clicking on edittext in Android Studio?
- AndroidX: ExampleInstrumentedTest.java Class Source code
- How to add user registration data into the Firebase database?
- Cannot find Symbol: ApplicationTestCase
- How do I use Android vector images in Android apps?
- How to create a new Android Virtual Device (AVD) in Android Studio?
- On SDK version 23 and up, your app data will be automatically backed up and restored on app install.
- App is not indexable by Google Search; consider adding atleast one Activity with an Action-View intent-filter.
- How do I style my button in Android app?
- How do I create drawable folder in Android Studio?
- How do I create new classes in Android Studio?
- How to create new android project tutorial?
- How to upgrade Android Studio 2.2.3 to new versions or Android Studio 3.5.3?
- error: Package R does not exist after renamed package name?