In this tutorial, I am going to show you how to add user registration data into the firebase database. Make sure you follow all my process and leave a comment below, if I did not explain in details.
Step 1: Connect Firebase
First of all, you need to connect your Android project to the firebase and add the required dependencies. If you don’t know how can you connect your project to a firebase, read this tutorial first which shows a firebase connection process.
Step 2: Connect Firebase Database
Click on the Tools -> Firebase to connect Firebase database like Firebase Authentication.
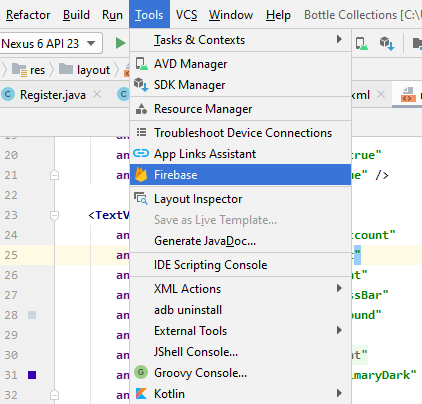
It will open the Firebase tab like the below image. Click on Realtime Database -> Get Started with Realtime Database -> Add Database. Follow the process to complete.
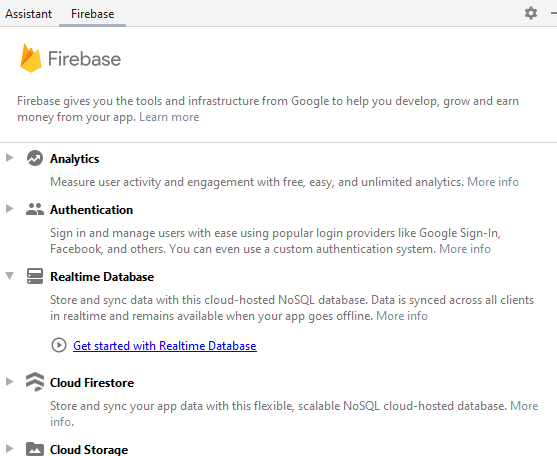
Now, you can open your Firebase console. Click on Realtime Database.
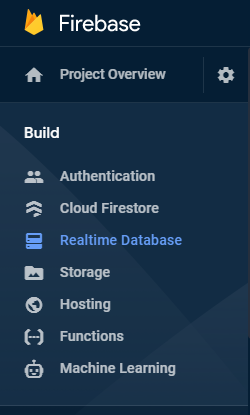
You will see an empty database with the root node and null value.
Click on rules, you need to change the Firebase database rules and allow only authenticated users to read and write data. So, replace the old Firebase rules with these new rules.
{
"rules": {
"users": {
"$userId": {
// grants write access to the owner of this user account
// whose uid must exactly match the key ($userId)
".read": "auth != null && $userId === auth.uid",
".write": "auth != null && $userId === auth.uid",
}
}
}
}
Step 3: Create User.java class
Now, lets go to the coding part. You have already created an authenticated registration form from step 1 tutorial. Now, this time we are going to add that data to the Firebase Database.
First, create a separate User.java class and generate getter and setter of the data, and create constructors. See a code below User.java.
package com.elsebazaar.bottlecollections;
public class User {
public String name;
public String email;
public String password;
public User(){
}
public User(String name, String email, String password){
this.name = name;
this.email = email;
this.password = password;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
Step 4: Add code to Register.java
This below code is used to add data to the Firebase
FirebaseDatabase firebaseDatabase = FirebaseDatabase.getInstance();
DatabaseReference databaseReference = firebaseDatabase.getReference();//databasereference
String uid = currentUser.getUid();
DatabaseReference userRef = databaseReference.child("users");//Create child node reference
userRef.child(uid).setValue(user);//Insert value to child node
Here is a complete code using during registration. The data should only add to the Firebase database after authentication success and user clicked the register button. So, the above code is added inside the register button onClicked method.
package com.elsebazaar.bottlecollections;
import android.content.Intent;
import android.graphics.Color;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ProgressBar;
import android.widget.TextView;
import androidx.annotation.NonNull;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.android.material.snackbar.Snackbar;
import com.google.firebase.auth.AuthResult;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.auth.FirebaseUser;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
public class Register extends MainActivity {
private FirebaseDatabase firebaseDatabase;
private DatabaseReference databaseReference;
private FirebaseAuth mAuth;
private User user;
ProgressBar progressBar;
EditText mName, mEmail, mPassword, mConfirmPassword;
Button btnregister;
TextView txtalreadyamember;
Snackbar snackbar;
String emailPattern = "[a-zA-Z0-9._-]+@[a-z]+\\.+[a-z]+";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.register);
progressBar = findViewById(R.id.progressBar);
mName = findViewById(R.id.ettxtName);
mEmail = findViewById(R.id.ettxtEmail);
mPassword = findViewById(R.id.ettxtPassword);
mConfirmPassword = findViewById(R.id.ettxtConfirmPassword);
btnregister = findViewById(R.id.btnRegister);
txtalreadyamember = findViewById(R.id.txtAlreadyAMember);
mAuth = FirebaseAuth.getInstance();
//initListeners();
btnregister.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = mName.getText().toString().trim();
String email = mEmail.getText().toString().trim();
String password = mPassword.getText().toString().trim();
String confirmpassword = mConfirmPassword.getText().toString().trim();
//check whether name is empty or not
if(TextUtils.isEmpty(name)){
mName.setError("Name is required");
return;
}
//check whether email address is empty or not
if(TextUtils.isEmpty(email)){
mEmail.setError("Email Address is required");
return;
}
//check email address pattern is correct or not
//if (isEmailValid(email))
if (!email.matches(emailPattern)){
mEmail.setError("Email Address is invalid");
return;
}
//check whether password is empty or not
if(TextUtils.isEmpty(password)){
mPassword.setError(" Password is required");
return;
}
//check whether password is less than 6 characters?
if(password.length() < 6){
mPassword.setError("Password must be >= 6 characters");
return;
}
//check whether password and confirm password are match or not
if(!password.equals(confirmpassword)){
mConfirmPassword.setError("Password does not match");
return;
}
//Make progress bar visible
progressBar.setVisibility(View.VISIBLE);
//User Method call
user = new User (name, email, password);
//create user by calling registerUser function
registerUser(email, password);
//Call function to empty All EditText
emptyInputEditText();
}
});
}
private void registerUser(String email, String password) {
//Register the user in firebase
mAuth.createUserWithEmailAndPassword(email,password).addOnCompleteListener(new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if (task.isSuccessful()){
FirebaseUser user = mAuth.getCurrentUser();
updateUI(user);
//display snackbar with green background
View view = findViewById(android.R.id.content);
String message = "Account Created Successfully";
int duration = Snackbar.LENGTH_LONG;
snackbar = Snackbar.make(view, message, duration)
.setAction("Action", null);
View sbView = snackbar.getView();
sbView.setBackgroundColor(Color.GREEN);
snackbar.show();
//Toast.makeText(Register.this,"Account Created",Toast.LENGTH_SHORT).show();
startActivity(new Intent(getApplicationContext(), Home.class));
}
else{
//display snackbar with red background
View view = findViewById(android.R.id.content);
String message = "Email ID already exits";
int duration = Snackbar.LENGTH_LONG;
snackbar = Snackbar.make(view, message, duration)
.setAction("Action", null);
View sbView = snackbar.getView();
sbView.setBackgroundColor(Color.RED);
snackbar.show();
//Toast.makeText(Register.this,"Error Occured" + task.getException(),Toast.LENGTH_SHORT).show();*/
// updateUI(null);
progressBar.setVisibility(View.INVISIBLE);
}
}
});
}
/**
* This method is to empty all input edit text
*/
private void emptyInputEditText() {
mName.setText(null);
mEmail.setText(null);
mPassword.setText(null);
mConfirmPassword.setText(null);
}
public void onStart(){
super.onStart();
//check if user is signed in and update UI accordingly
FirebaseUser currentUser = mAuth.getCurrentUser();
updateUI(currentUser);
}
private void updateUI(FirebaseUser currentUser) {
if (user != null) {
firebaseDatabase = FirebaseDatabase.getInstance();
databaseReference = firebaseDatabase.getReference();//databasereference
String uid = currentUser.getUid();
DatabaseReference userRef = databaseReference.child("users");//Create child node reference
userRef.child(uid).setValue(user);//Insert value to child node
}
}
public void enabled(View view) {
Intent intent = new Intent(Register.this, MainActivity.class);
startActivity(intent);
finish();
}
}
That's it. Run your program and see the result.
You may interest on the following topics:
- How to implement searchView widget in android Studio through Recycleview?
- How to create an expandable listview in Fragments in Android Studio?
- How to create an expandable list view in Android Studio?
- How do I check Intel Virtualization Technology is enabled or not in Windows 10?
- Plugin ‘Android WiFi ADB’ is compatible with IntelliJ IDEA only because it doesn’t define any explicit module dependencies
- Could not install Gradle Distribution from ‘https://services.gradle.org/distributions/gradle-6.5-all.zip’
- How to solve “INSTALL_PARSE_FAILED_NO_CERTIFICATES” error in Android Studio?
- Android Studio Run/Debug configuration error: Module not specified.
- How to search in multiple nodes in Firebase Database?
- How to get the sum of child nodes in Firebase database?
- How to display website in an android app using WebView component?
- Android Layout Using ViewPager and Fragments
- How do I install Android Studio in Windows 10?
- How to display ListView in Fragments using ViewPagers?
- How to create a custom AlertDialog in Android Studio?
- How do I change the name under apps that display in google play store?
- Where does my database store in Android Studio?
- How to add google places autocomplete in Android Edittext?
- How do I convert dp into px in the android studio?
- What are the android screen background sizes?
- What are the sizes of the Android icon located inside the mipmap?
- How do I remember my android app signing key?
- How do I create a Toolbar in Android Studio?
- How to get Android Spinner Dropdown?
- error: package R doesn’t exist in android studio project?
- Firebase Email and Password registration for Android App
- How do I change the company domain name in Android Studio after creating a project?
- How do I make an existing Android Studio Project copy?
- How do I migrate an Android Studio 3.2 existing project to Android X?
- Step by step to insert both banner and interstitial ads in android apps.
- Android TimePicker upon clicking on edittext in Android Studio?
- Migrating to AndroidX Errors:
- How to popup date picker when clicking on edittext in Android Studio?
- AndroidX: ExampleInstrumentedTest.java Class Source code
- How to add user registration data into the Firebase database?
- Cannot find Symbol: ApplicationTestCase
- How do I use Android vector images in Android apps?
- How to create a new Android Virtual Device (AVD) in Android Studio?
- On SDK version 23 and up, your app data will be automatically backed up and restored on app install.
- App is not indexable by Google Search; consider adding atleast one Activity with an Action-View intent-filter.
- How do I style my button in Android app?
- How do I create drawable folder in Android Studio?
- How do I create new classes in Android Studio?
- How to create new android project tutorial?
- How to upgrade Android Studio 2.2.3 to new versions or Android Studio 3.5.3?
- error: Package R does not exist after renamed package name?