Android ExpandableListView is a view that shows an item in a vertically scrolling two-level list. It is expanding and collapses whenever the user touches on a group item. The top-level list is group items and second-level items are their respective child items.
Today, I am going to show you how to create expandable ListView exactly the same as shown in the below video.
Step to follow:
- Create your expandableListView layout in activity_xml file
- Create header items layout file and name as header_item.xml
- Create Child items layout file and name as child_item.xml
- Create a Custom Expandable ListView Adapter in Java class and name as customexpandablelistviewadapter.java
- Add some code in your MainActivity.java
Here is a screenshot how your above files look like after being created.
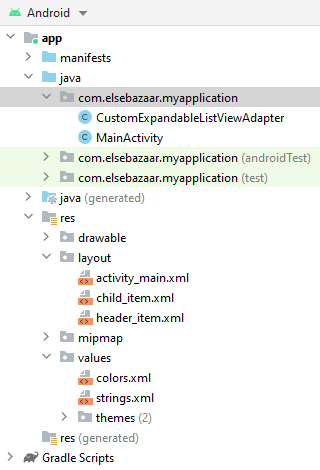
Now let’s start the above steps from 1 to 5.
1) activity_main.xml
Design your expandable listview layout inside this activity_main.xml file. Add the below code.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:ads="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorAccent"
android:padding="10dp">
<ExpandableListView
android:id="@+id/expListView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorAccent"
android:divider="@android:color/holo_blue_dark"
android:dividerHeight="1dp"/>
</RelativeLayout>
2) header_item.xml
Create another XML file for your group header titles and name as header_item.xml and add the following code inside it.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/title"
android:layout_width="match_parent"
android:layout_height="40dp"
android:textSize="16sp"
android:gravity="center_vertical"
android:textColor="@color/black"
android:text="I am a Header Group Item" />
</LinearLayout>
3) child_item.xml
Create another child layout for child items and name as child_item.xml. Design your child’s layout as you like. Here is a sample of my child_item.xml layout.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/expandedChildItems"
android:layout_width="match_parent"
android:layout_height="30dp"
android:textSize="16sp"
android:gravity="center_vertical"
android:textColor="@color/black"
android:divider="@android:color/holo_blue_dark"
android:dividerHeight="1dp"
android:text="I am child items" />
</LinearLayout>
4) Custom Expandable ListView Adapter
Create your custom expandable listview adapter and name it as CustomExpandableListViewAdapter.java and extends this adapter from BaseExpandableListAdapter.
Here is a full code of my CustomExpandableListViewAdapter.java class
package com.elsebazaar.myapplication;
import android.content.Context;
import android.graphics.Typeface;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseExpandableListAdapter;
import android.widget.TextView;
import java.util.HashMap;
import java.util.List;
import java.util.Objects;
public class CustomExpandableListViewAdapter<S> extends BaseExpandableListAdapter {
private final Context context;
private final List<String> headerItem;
private final HashMap<String, List<String>> childItem;
public CustomExpandableListViewAdapter(Context context, List<String> headerItem, HashMap<String, List<String>> childItem) {
this.context = context;
this.headerItem = headerItem;
this.childItem = childItem;
}
@Override
public int getGroupCount() {
return this.headerItem.size();
}
@Override
public int getChildrenCount(int groupPosition) {
return Objects.requireNonNull(this.childItem.get(this.headerItem.get(groupPosition))).size();
}
@Override
public Object getGroup(int groupPosition) {
return this.headerItem.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
return Objects.requireNonNull(this.childItem.get(this.headerItem.get(groupPosition))).get(childPosition);
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
@Override
public boolean hasStableIds() {
return true;
}
@Override
public View getGroupView(final int groupPosition, boolean isExpanded, View convertView, ViewGroup parentView) {
String headerTitle = getGroup(groupPosition).toString();
if(convertView == null) {
LayoutInflater inflater = (LayoutInflater) this.context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
assert inflater != null;
convertView = inflater.inflate(R.layout.header_item, parentView, false);
}
TextView txtHeader = convertView.findViewById(R.id.title);
txtHeader.setTypeface(null, Typeface.BOLD);
txtHeader.setText(headerTitle);
return convertView;
}
@Override
public View getChildView(int groupPosition, final int childPosition, boolean isLastChild, View convertView, ViewGroup parentView) {
final String expandedChildList = (String) getChild(groupPosition, childPosition);
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
assert inflater != null;
convertView = inflater.inflate(R.layout.child_item,parentView,false);
}
TextView txtChildItems = convertView.findViewById(R.id.expandedChildItems);
txtChildItems.setText(expandedChildList);
return convertView;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return false;
}
@Override
public void notifyDataSetChanged() {
super.notifyDataSetChanged();
}
}
5) MainActivity.java
Once you have done creating your custom expandable listview adapter. Open your MainActivity.java class and make the changes you have seen in the below code.
package com.elsebazaar.myapplication;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.ExpandableListView;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
public class MainActivity extends AppCompatActivity {
ExpandableListView expandableListView;
CustomExpandableListViewAdapter adapter;
List<String> listDataHeader;
HashMap<String, List<String>> listDataChild;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//initialize variable
expandableListView = findViewById(R.id.expListView);
// preparing list data
prepareListData();
adapter = new CustomExpandableListViewAdapter(this, listDataHeader, listDataChild);
expandableListView.setAdapter(adapter);
}
private void prepareListData() {
listDataHeader = new ArrayList<String>();
listDataChild = new HashMap<String, List<String>>();
// Adding child data
listDataHeader.add("Fruits");
listDataHeader.add("Vegetables");
listDataHeader.add("Meats");
listDataHeader.add("Drinks");
// Adding child data
List<String> Fruits = new ArrayList<String>();
Fruits.add("Apples");
Fruits.add("Bananas");
Fruits.add("Mangoes");
Fruits.add("Pineapples");
Fruits.add("Watermelon");
Fruits.add("Dragon Fruits");
Fruits.add("Kiwi");
List<String> Vegetables = new ArrayList<String>();
Vegetables.add("Cabbage");
Vegetables.add("Brussels Sprouts");
Vegetables.add("Carrots");
Vegetables.add("Collard Greens");
Vegetables.add("Eggplant");
Vegetables.add("Cauliflower");
List<String> Meats = new ArrayList<String>();
Meats.add("Beef");
Meats.add("Lamb");
Meats.add("Veal");
Meats.add("Pork");
Meats.add("Kangaroo");
List<String> Drinks = new ArrayList<String>();
Drinks.add("Coca Cola");
Drinks.add("Fanta");
Drinks.add("Sprite");
Drinks.add("Soda");
Drinks.add("Lemonade");
listDataChild.put(listDataHeader.get(0), Fruits); // Header, Child data
listDataChild.put(listDataHeader.get(1), Vegetables);
listDataChild.put(listDataHeader.get(2), Meats);
listDataChild.put(listDataHeader.get(3), Drinks);
}
}
Output:
You may be interested in the following topics:
- How to implement searchView widget in android Studio through Recycleview?
- How to create an expandable listview in Fragments in Android Studio?
- How to create an expandable list view in Android Studio?
- How do I check Intel Virtualization Technology is enabled or not in Windows 10?
- Plugin ‘Android WiFi ADB’ is compatible with IntelliJ IDEA only because it doesn’t define any explicit module dependencies
- Could not install Gradle Distribution from ‘https://services.gradle.org/distributions/gradle-6.5-all.zip’
- How to solve “INSTALL_PARSE_FAILED_NO_CERTIFICATES” error in Android Studio?
- Android Studio Run/Debug configuration error: Module not specified.
- How to search in multiple nodes in Firebase Database?
- How to get the sum of child nodes in Firebase database?
- How to display website in an android app using WebView component?
- Android Layout Using ViewPager and Fragments
- How do I install Android Studio in Windows 10?
- How to display ListView in Fragments using ViewPagers?
- How to create a custom AlertDialog in Android Studio?
- How do I change the name under apps that display in google play store?
- Where does my database store in Android Studio?
- How to add google places autocomplete in Android Edittext?
- How do I convert dp into px in the android studio?
- What are the android screen background sizes?
- What are the sizes of the Android icon located inside the mipmap?
- How do I remember my android app signing key?
- How do I create a Toolbar in Android Studio?
- How to get Android Spinner Dropdown?
- error: package R doesn’t exist in android studio project?
- Firebase Email and Password registration for Android App
- How do I change the company domain name in Android Studio after creating a project?
- How do I make an existing Android Studio Project copy?
- How do I migrate an Android Studio 3.2 existing project to Android X?
- Step by step to insert both banner and interstitial ads in android apps.
- Android TimePicker upon clicking on edittext in Android Studio?
- Migrating to AndroidX Errors:
- How to popup date picker when clicking on edittext in Android Studio?
- AndroidX: ExampleInstrumentedTest.java Class Source code
- How to add user registration data into the Firebase database?
- Cannot find Symbol: ApplicationTestCase
- How do I use Android vector images in Android apps?
- How to create a new Android Virtual Device (AVD) in Android Studio?
- On SDK version 23 and up, your app data will be automatically backed up and restored on app install.
- App is not indexable by Google Search; consider adding atleast one Activity with an Action-View intent-filter.
- How do I style my button in Android app?
- How do I create drawable folder in Android Studio?
- How do I create new classes in Android Studio?
- How to create new android project tutorial?
- How to upgrade Android Studio 2.2.3 to new versions or Android Studio 3.5.3?
- error: Package R does not exist after renamed package name?