This article will show you how can you implement google places API in your app. Google made an autocomplete places API, which makes it easier to select an address and also gives a suggestion when you start typing.
Let’s begin with how to implement google places API in your app. Here are the shortlists of step I am going to explain:
- Google Developer Console
- Strings.xml
- XML File
- Java Code
- Output
1) Google Developer Console
Here, you have to enable Google Places API and create credentials. Lets begin.
1.1) login
First, login to your google developer console. Click on “Credentials” on the left-hand sidebar.

1.2) CREATE CREDENTIALS
Now, add your new credential for your project by clicking “CREATE CREDENTIALS“. After that click on the edit button to change your API credential name and restriction mode and save your API credential.
You also need to add your package name and SHA-1 fingerprint. You can find package name on the top of your each java file.
package com.elsebazaar.googleplaces;
SHA-1 fingerprint can be found in your project. Go to Gradle ->Your Project Name ->Tasks ->Android ->signingReport. Check your project log, your project SHA-1 fingerprint is there.
1.3) Enable Google Places API
Go to Library -> Place API and enable your Google Places API.
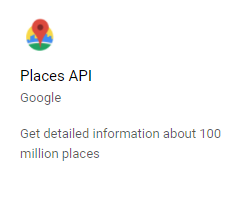
1.4) Copy API Key
You have to use your API key in your project. To get your API key, go back to credential again and select your app credential. You will see an API KEY located on the right-hand side. Copy your API key for now.
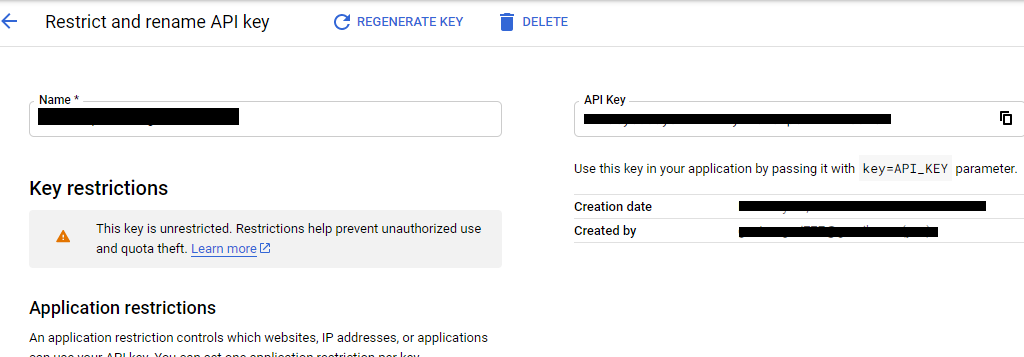
2) String.xml
Create a resource of your API key and paste the above API key value in “Your_API_KEY”.
<resources>
<string name="api_key">Your_API_KEY</string>
</resources>
3) XML File
Create your .xml file with one edittext. An example is shown below.
<EditText
android:id="@+id/etaddress"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/white"
android:textColor="@color/black"
android:autofillHints="@string/hint_address"
android:hint="@string/hint_address"
android:ems="10"
android:inputType="textPostalAddress"
android:layout_weight="1"/>
4) Java Code
Implement two libraries in your project gradle
implementation 'com.google.android.gms:play-services-location:17.1.0'
implementation 'com.google.android.libraries.places:places:2.4.0'
4.1) Initialize edittext
EditText maddress;
4.2) Assign edittext
maddress = findViewById(R.id.etaddress);
4.3) Initialize Places
String apiKey = getString(R.string.api_key);
if (!Places.isInitialized()) {
Places.initialize(getApplicationContext(), apiKey);
}
4.4) edittext setOnClickListerner
//maddress on clicklistener
maddress.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Set the fields to specify which types of place data to
// return after the user has made a selection.
List<Place.Field> field = Arrays.asList(Place.Field.ID, Place.Field.ADDRESS);
// Start the autocomplete intent.
Intent intent = new Autocomplete.IntentBuilder(AutocompleteActivityMode.FULLSCREEN, field)
.build(Address.this);
//start activity result
startActivityForResult(intent, AUTOCOMPLETE_REQUEST_CODE);
}
});
4.5) Result Activity
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == AUTOCOMPLETE_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
//When success initialize place
Place place = Autocomplete.getPlaceFromIntent(data);
//set address on edittext
maddress.setText(place.getAddress());
} else if (resultCode == AutocompleteActivity.RESULT_ERROR) {
// TODO: Handle the error.
Status status = Autocomplete.getStatusFromIntent(data);
//Log.i(TAG, status.getStatusMessage());
} else if (resultCode == RESULT_CANCELED) {
// The user canceled the operation.
}
}
}
5) Output
Run your program and see the output
You may interest on the following topics:
- How to implement searchView widget in android Studio through Recycleview?
- How to create an expandable listview in Fragments in Android Studio?
- How to create an expandable list view in Android Studio?
- How do I check Intel Virtualization Technology is enabled or not in Windows 10?
- Plugin ‘Android WiFi ADB’ is compatible with IntelliJ IDEA only because it doesn’t define any explicit module dependencies
- Could not install Gradle Distribution from ‘https://services.gradle.org/distributions/gradle-6.5-all.zip’
- How to solve “INSTALL_PARSE_FAILED_NO_CERTIFICATES” error in Android Studio?
- Android Studio Run/Debug configuration error: Module not specified.
- How to search in multiple nodes in Firebase Database?
- How to get the sum of child nodes in Firebase database?
- How to display website in an android app using WebView component?
- Android Layout Using ViewPager and Fragments
- How do I install Android Studio in Windows 10?
- How to display ListView in Fragments using ViewPagers?
- How to create a custom AlertDialog in Android Studio?
- How do I change the name under apps that display in google play store?
- Where does my database store in Android Studio?
- How to add google places autocomplete in Android Edittext?
- How do I convert dp into px in the android studio?
- What are the android screen background sizes?
- What are the sizes of the Android icon located inside the mipmap?
- How do I remember my android app signing key?
- How do I create a Toolbar in Android Studio?
- How to get Android Spinner Dropdown?
- error: package R doesn’t exist in android studio project?
- Firebase Email and Password registration for Android App
- How do I change the company domain name in Android Studio after creating a project?
- How do I make an existing Android Studio Project copy?
- How do I migrate an Android Studio 3.2 existing project to Android X?
- Step by step to insert both banner and interstitial ads in android apps.
- Android TimePicker upon clicking on edittext in Android Studio?
- Migrating to AndroidX Errors:
- How to popup date picker when clicking on edittext in Android Studio?
- AndroidX: ExampleInstrumentedTest.java Class Source code
- How to add user registration data into the Firebase database?
- Cannot find Symbol: ApplicationTestCase
- How do I use Android vector images in Android apps?
- How to create a new Android Virtual Device (AVD) in Android Studio?
- On SDK version 23 and up, your app data will be automatically backed up and restored on app install.
- App is not indexable by Google Search; consider adding atleast one Activity with an Action-View intent-filter.
- How do I style my button in Android app?
- How do I create drawable folder in Android Studio?
- How do I create new classes in Android Studio?
- How to create new android project tutorial?
- How to upgrade Android Studio 2.2.3 to new versions or Android Studio 3.5.3?
- error: Package R does not exist after renamed package name?